Use PyPDF2 - Merging multiple pages and adding watermarks
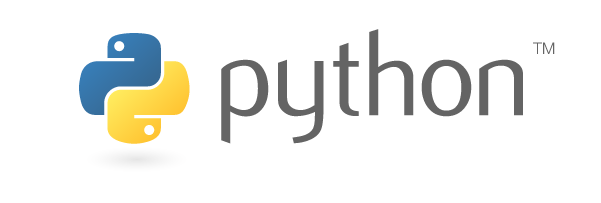
In the following article I wrote previously, I was able to use PyPDF2 to extract text information from PDF files.
In this article we will use the page merging feature of PyPDF2 to achieve a way to put a “watermark” in the file.
Goals
- Use PyPDF2
- Add a common “watermark” to the file.
Preparation
As before, we will use the file downloaded from the Executive Orders.
It looks like the following. There are about three pages in total. Save it as executive_order.pdf
.
Next, prepare a PDF file for the “watermark”. Save it as confidential.pdf
.
Merging PDF files
Let’s merge the two files with PyPDF2. The sample code now looks like the following
1import PyPDF2
2
3FILE_PATH = './files/executive_order.pdf'
4CONFIDENTIAL_FILE_PATH = './files/confidential.pdf'
5OUTPUT_FILE_PATH = './files/executive_order_merged.pdf'
6
7with open(FILE_PATH, mode='rb') as f, open(CONFIDENTIAL_FILE_PATH, mode='rb') as cf, open(OUTPUT_FILE_PATH, mode='wb') as of:
8 conf_reader = PyPDF2.PdfFileReader(cf)
9 conf_page = conf_reader.getPage(0)
10 reader = PyPDF2.PdfFileReader(f)
11 writer = PyPDF2.PdfFileWriter()
12 for page_num in range(0, reader.numPages):
13 obj = reader.getPage(page_num)
14 obj.mergePage(conf_page)
15
16 writer.addPage(obj)
17
18 writer.write(of)
I’ll explain it briefly, step by step.
First, get the first page from the confidential.pdf
file with getPage(0)
to get the first page with the “watermark” printed on it.
Next, call the mergePage
function on all page objects in the executive_order.pdf
file.
Then, merge the watermark page that you have just obtained.
Finally, we will use PdfFileWriter
to write in a file with the alias executive_order_merged.pdf
.
Check the results
It’s watermarked properly. In this sample code, all three pages are watermarked.
Conclusion
In this article we have shown how to merge PDF pages using PyPDF2.
This is very easy to achieve since you only need to use the mergePage
function.
I’m sure there are situations where it could be used, such as inserting a common footer or signature into a page.