HTTP requests in Python3 with Requests library
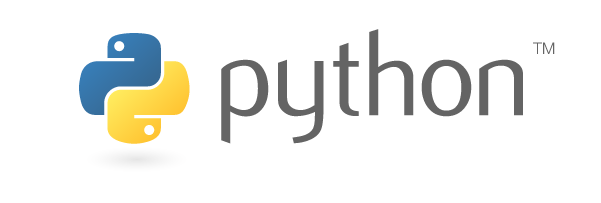
In past article “HTTP requests in Python3 with urllib.request module”, I wrote simple code samples for HTTP requests with urllib.request module which is a package in Python.
In this article, I introduce Requests.
What is Requests
Requests is a package for HTTP request.
Requests is a human readable package for HTTP request with wrapping urllib
.
It has a simple interface and supports lots of feature as follows.
- International Domains and URLs
- Keep-Alive & Connection Pooling
- Sessions with Cookie Persistence
- Browser-style SSL Verification
- Basic/Digest Authentication
- Elegant Key/Value Cookies
- Automatic Decompression
- Automatic Content Decoding
- Unicode Response Bodies
- Multipart File Uploads
- HTTP(S) Proxy Support
- Connection Timeouts
- Streaming Downloads
- .netrc Support
- Chunked Requests
Setup
Install Requests with pip
.
1pip install requests
HTTP request with Requests package
First, try to request with HTTP GET.
1import requests
2r = requests.get('https://github.com/timeline.json')
That’s is very simple code. Awsome.
You can do request other HTTP method (POST
and PUT
etc..) as well.
If request body needed, give data
option.
1import requests
2import json
3r = requests.post('xxxxxxxx', data=json.dumps({'hoge':'huga'}))
4r = requests.put('xxxxxxx', data=json.dumps({'hoge':'huga'}))
Response handling
If the response data is json format, convert data to json with json()
function.
1import requests
2r = requests.get('https://github.com/timeline.json')
3r.json()
However, json()
function returns None
when it failed to decode.
So I recommend to give text
property in response object to json.loads
function as follows if you’d like to handle as error in this case.
1import json
2import requests
3
4try:
5 r = requests.get('https://github.com/timeline.json')
6 res = json.loads(r.text)
7except json.JSONDecodeError as e:
8 print(e)
Get the response status code in HTTP redirect
Response object has a status_code
property. However, this status code means “a status code for the last request”.
In other words, we can’t get status code 3xx
in this way.
We can access to redirected response data with history
property in response object when HTTP redirect occured.
These data are sorted in order from the old and stored.
1r = requests.get('http://github.com')
2his = r.history
3print(his[0].status_code) # 301
Conclusion
It is available to
- Request with Requests
- Get HTTP status code when HTTP redirect occured