Use Range function in Python
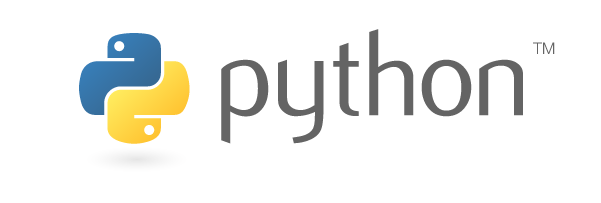
Python has more utility function implementations than other programming languages.
This time, I will introduce the for statement processing using the Python range()
function.
Environment
I have confirmed the operation in the following environment.
- Python 3.8
range() function is
A range ()
is a Python built-in function used to create a sequence of numbers and mainly used in combination with the for statement. Takes several arguments to control the rules for generating sequence.
Use range() function
Generate a sequence starting from 0
This is the most basic usage. Give an integer argument to the range ()
function.
In following example, generate a sequence of numbers starting from 0
up to number - 1
given an integer argument to range () function.
1for i in range(5):
2 print(i)
3
4> 0
5> 1
6> 2
7> 3
8> 4
Generate a sequence starting with an arbitrary number
By giving two integer arguments to the range ()
function, it will generate a sequence of numbers starting from number of first argument
up to number of second argument-1
.
1for i in range(2, 5):
2 print(i)
3
4> 2
5> 3
6> 4
range (0, N)
is equivalent to range (N)
(N
is any integer value).
1for i in range(0, 5):
2 print(i)
3
4> 0
5> 1
6> 2
7> 3
8> 4
The value of the first argument must be smaller than the value of the second argument. This is because it adds one by one implicitly internally. An example of no sequence generated is as follows.
1for i in range(10, 0):
2 print(i)
3
4>
Generate a sequence of numbers starting with any number and with any addition step
If you give three integer arguments to the range ()
function, generate the number of sequence starting with the number of the first argument
and ending with the number of the second argument -1
in number increments of the third argument
.
In the following, a number sequence is made by adding 2 between integer values from 2 to 8.
1for i in range(2, 8, 2):
2 print(i)
3
4> 2
5> 4
6> 6
Generate a sequence of decreasing numbers by giving a negative integer to the third argument.
1for i in range(5, 0, -1):
2 print(i)
3
4> 5
5> 4
6> 3
7> 2
8> 1
Unfortunately, it is not possible to generate a sequence that handles a decimal fraction.
1for i in range(1, 5, 0.2):
2 print(i)
3
4> TypeError: 'float' object cannot be interpreted as an integer
Use it with list comprehensions
Initialize a numeric array by combining the range ()
function with a list comprehension.
1arr = [i for i in range(1, 10, 3)]
2print(arr)
3
4> [1, 4, 7]
Advantages of using the range () function
The object created by the range ()
function is not an array type , but a range
class type . Print the object type.
1hoge = range(1, 10)
2print(hoge)
3
4> range(1, 10)
5
6print(type(hoge))
7
8> <class 'range'>
That is stated in the Official Python documents. The following is a quote.
In many ways the object returned by range() behaves as if it is a list, but in fact it isn’t. It is an object which returns the successive items of the desired sequence when you iterate over it, but it doesn’t really make the list, thus saving space.