Python3でHTTP通信をする(urllib.requestモジュールを使う)
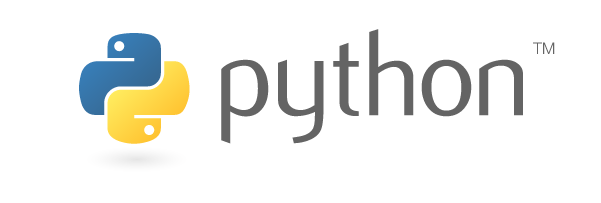
目次
今回はPythonでHTTP通信を行うためのモジュールである urllib.request モジュールを紹介します。
環境情報
今回は以下の環境にて動作確認をしています。
- python 3.7
基本的な通信
Request オブジェクトを使ったGET
urllib.request
モジュール内に含まれる Request
オブジェクトを使います。
以下のようにインスタンスを作成します。
1from urllib.request import *
2
3req = Request(url='https://docs.python.org/ja/', headers={}, method='GET')
Request
オブジェクトのインスタンスのプロパティにアクセスしてみましょう。
オリジンの情報やパスなどの情報が取得できることがわかります。
1print(req.full_url)
2> https://docs.python.org/ja/
3
4print(req.type)
5> https
6
7print(req.host)
8> docs.python.org
9
10print(req.origin_req_host)
11> docs.python.org
12
13print(req.selector)
14> /ja/
15
16print(req.data)
17> None
18
19print(req.unverifiable)
20> False
21
22print(req.method)
23> GET
あとはこれを urlopen
関数で処理してあげるだけです。
1with urlopen(req) as res:
2 body = res.read().decode()
3 print(body)
Request オブジェクトを使ったPOST
今度は POST
で送信します。
リクエストボディを指定するには Request
オブジェクトのインスタンス生成時に data
オプションを指定してします。
1from urllib.request import *
2import json
3
4req = Request(url='https://docs.python.org/ja/', data=json.dumps({'param': 'piyo'}).encode(), headers={}, method='POST')
5
6with urlopen(req) as res:
7 body = res.read().decode()
8 print(body)
TypeError: can't concat str to bytes
が表示されるときは data
に渡すオブジェクトがエンコードされていないことが原因です。
上の例では json.dumps({'param': 'piyo'}).encode()
で辞書型をjsonにエンコードしています。
urlopen
だけで通信する
先程のような簡単な通信であれば Request
オブジェクトを使わなくても urlopen
関数単体で実現できます。
1from urllib.request import *
2
3with urlopen('https://docs.python.org/ja/') as res:
4 body = res.read().decode()
5 print(body)