Step FunctionsでCloudWatch LogsのロググループをS3へエクスポートする
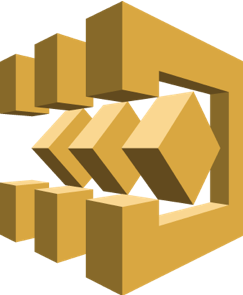
CloudWatch LogsにはロググループをS3にエクスポートする機能がついています。 しかし、エクスポート機能には同時実行数制限があるので、 今回は Step Functions を使ってS3へのログのエクスポートを実現しました。
モチベーション
ログをS3にエクスポートしたい
CloudWatch Logsのコンソールは特定の文字列を含むログを検索するのは得意ですが、ログの集計や可視化には向いていません。 S3にログを集積し、そこをDWHにしてETL処理を施して可視化したりするのが事例としてよく見かけるので、 今回は Cloudwatch LogsのいくつかのログストリームをS3にエクスポートしたい と考えました。
Subscription Filterが使われている、、だと!?
CloudWatch LogsからS3へログストリームを転送する簡易な方法は Kinesis Firehose を使う方法です。
リアルタイムでログを流し込めますし、処理中に Lambda 関数を挟むことができるので大変便利ですが、ここで一つ問題がありました。
それは 既に Subscription Filter が埋まっていた ことです。
(上は既にKinesis StreamにSubscription Filterを奪われた図)
CloudWatch Logsを外部のAWSリソースにストリーム接続させるには Subscription Filter
を設定する必要があるのですが、仕様上、 1ログストリームに対して1Subscription Filterしか設定できません。
大抵、CloudWatch Logsの重要なログストリームほど、Subscription Filterが既に何者かによって設定されていて、運用上すんなり引っ剥がせないのです。
Create Export Taskは同時に1実行まで
そこで、CloudWatch Logsの ログストリームをS3にエクスポートする機能 を使おうと考えるわけです。
エクスポートする時間帯をUTCでレンジ指定することで、対象期間のログストリームを任意のバケットにエクスポートする タスク を登録できます。
ただこれにも制約があります。エクスポート機能は1度に1回しか実行できない のです。
エクスポートするためのタスクを生成した後、そのエクスポートタスクのステータスが COMPLETED
になるまで、
次のエクスポートを設定できません。
実装目線で言えば、エクスポートタスクの終了を待ち合わせて、次のログストリームをエクスポートタスクを設定する 必要がありそうです。
Step Functionsで create export task APIを呼びまくる
というわけでやってみましょう。今回実装した処理の流れはざっくり以下です。
- CloudWatch Events で定期的に(Dailyとか) Step Functions を実行
- Step Functionsから Lambda を キック
- Lambda では CloudWatch Logs の create export task を実行
- create export task で S3にログがエクスポートされる
順を追って見ていきましょう。手順的には後段から作る必要があるので、それに準じて説明します。
S3バケットを準備する
まずは、ログがエクスポートされるS3バケットを作成しましょう。
バケットは普通に作れば良いとして、 バケットポリシーを以下のように指定する のがポイントです。
1{
2 "Version": "2012-10-17",
3 "Statement": [
4 {
5 "Sid": "1",
6 "Effect": "Allow",
7 "Principal": {
8 "Service": "logs.${リージョン名}.amazonaws.com"
9 },
10 "Action": "s3:PutObject",
11 "Resource": "arn:aws:s3:::${バケット名}/*",
12 "Condition": {
13 "StringEquals": {
14 "s3:x-amz-acl": "bucket-owner-full-control"
15 }
16 }
17 },
18 {
19 "Sid": "2",
20 "Effect": "Allow",
21 "Principal": {
22 "Service": "logs.${リージョン名}.amazonaws.com"
23 },
24 "Action": "s3:GetBucketAcl",
25 "Resource": "arn:aws:s3:::${バケット名}"
26 }
27 ]
28}
Lambdaの実装
次に CloudWatch Logsのexport task apiをコールするためのLambda Functionを作成します。 以下にコードサンプルを載せます。
1#!/usr/bin/env python
2# -*- coding:utf-8 -*-
3
4import logging
5import boto3
6from datetime import datetime
7from datetime import timedelta
8from dateutil.parser import parse
9import pytz
10import time
11import os
12
13def _is_executing_export_tasks():
14 '''
15 export taskが実行中かどうかチェック
16 '''
17 client = boto3.client('logs')
18 for status in ['PENDING', 'PENDING_CANCEL', 'RUNNING']:
19 response = client.describe_export_tasks(limit = 50, statusCode=status)
20 if 'exportTasks' in response and len(response['exportTasks']) > 0:
21 return True
22 return False
23
24def _get_target_date(event):
25 '''
26 CloudWatch Eventsの(実行日時 - 1)日をエクスポート対象にする
27 '''
28 target = None
29 tokyo_timezone = pytz.timezone('Asia/Tokyo')
30
31 utc_dt = datetime.strptime(event['time'], '%Y-%m-%dT%H:%M:%SZ')
32 tokyo_time = utc_dt.astimezone(tokyo_timezone)
33 target = tokyo_time - timedelta(days=1)
34 t = target.replace(hour=0, minute=0, second=0, microsecond=0)
35
36 target_date = t.strftime('%Y%m%d')
37 from_time = int(t.timestamp() * 1000)
38 to_time = int((t + timedelta(days=1) - timedelta(milliseconds=1)).timestamp() * 1000)
39 return from_time, to_time, target_date
40
41def _get_log_group(next_token):
42 '''
43 ロググループを取得する
44 '''
45 client = boto3.client('logs')
46 if next_token is not None and next_token != '':
47 response = client.describe_log_groups(limit = 50, nextToken = next_token)
48 else:
49 # nextTokenは空文字を受け付けない
50 response = client.describe_log_groups(limit = 50)
51
52 if 'logGroups' in response:
53 yield from response['logGroups']
54 # ロググループが多くて50件(最大)を超えるようなら再帰呼出し
55 if 'nextToken' in response:
56 yield from _get_log_group(next_token = response['nextToken'])
57
58def _is_bucket_object_exists(bucket_name, bucket_dir):
59 client = boto3.client('s3')
60 response = client.list_objects_v2(Bucket = bucket_name, Prefix = (bucket_dir))
61 return 'Contents' in response and len(response['Contents']) > 0
62
63def _export_logs_to_s3(bucket_name, bucket_dir, from_time, to_time, log_group_name):
64 client = boto3.client('logs')
65 response = client.create_export_task(taskName = bucket_dir, logGroupName = log_group_name, fromTime = from_time, to = to_time, destination = bucket_name, destinationPrefix = bucket_dir)
66
67def lambda_handler(event, context):
68 bucket_name = os.environ['BUCKET_NAME']
69 from_time, to_time, target_date = _get_target_date(event=event)
70
71 if _is_executing_export_tasks():
72 return {
73 "status": "running",
74 "time": event['time']
75 }
76
77 for log_group in _get_log_group(next_token=None):
78 bucket_dir = log_group['logGroupName'] + '/' +target_date
79 if log_group['logGroupName'].find('/') == 0:
80 bucket_dir = log_group['logGroupName'][1:]
81
82 if _is_bucket_object_exists(bucket_name = bucket_name, bucket_dir = bucket_dir):
83 continue
84 _export_logs_to_s3(bucket_name = bucket_name, log_group_name = log_group['logGroupName'], from_time= from_time, to_time = to_time, bucket_dir = bucket_dir)
85 return {
86 "status": "running",
87 "time": event['time']
88 }
89
90 return {
91 "status": "completed",
92 "time": event['time']
93 }
ポイントは Lambdaに戻り値を設定する ことです。 今までLamdaで戻り値を指定しても使いみちは殆ど無かったのですが、ここでは役に立つのです。
Step Functionsでは戻り値を受け取り、それを後段のタスクに渡すことができます。
Step Functionsの実装
次にステートマシンを定義します。今回のメインはこれです。
定義したステートマシンは以下のようになっています。
各ステートの説明はざっくり以下になります。
Export Awslogs to S3
:create export taskをするFinished exporting?
:処理中または処理可能なログストリームが存在するかチェックするSuccess
:ステートマシンの終了Wait a minute
:export taskが終わりそうな時間を適当に待つ
1{
2 "Comment": "Export Cloudwatch LogStream recursively",
3 "StartAt": "Export Awslogs to S3",
4 "TimeoutSeconds": 86400,
5 "States": {
6 "Export Awslogs to S3": {
7 "Type": "Task",
8 "Resource": "${Lambdaのarn}",
9 "Next": "Finished exporting?"
10 },
11 "Finished exporting?": {
12 "Type": "Choice",
13 "Choices":[{
14 "Variable": "$.status",
15 "StringEquals": "running",
16 "Next": "Wait a minute"
17 },{
18 "Variable": "$.status",
19 "StringEquals": "completed",
20 "Next": "Success"
21 }]
22 },
23 "Wait a minute": {
24 "Type": "Wait",
25 "Seconds": 5,
26 "Next": "Export Awslogs to S3"
27 },
28 "Success": {
29 "Type": "Succeed"
30 }
31 }
32}
ポイントとしては、Waitのステートを入れている ところです。
create export task のAPIは同時に実行できないので、COMPLETE
するまでの概算秒数を入れています。
Lambdaには実行時間の上限があるのと、稼働時間に応じた課金になる ので、Lambda関数の中でsleepするのは好ましくありません。
もちろん、秒数は概算で問題なく、エクスポートが COMPLETE
以外の場合には
Export Awslogs to S3
→ Finished exporting?
→ Wait a minute
をぐるぐる回るようにしておくのが良いでしょう。
とは言え、無限ループにならないようにタイムアウト値 TimeoutSeconds: 86400
(24時間)を指定しています。
少し長いかもしれないので、要調整です。
CloudWatch Eventsの設定
CloudWatch Eventsでタイムベースのトリガー指定をします。
Step Functionsのarnを指定するだけなので、そこまで凝った所はありません。
このときに指定するロールには、信頼関係に events.amazonaws.com
を指定し、
最低限以下のポリシーが必要です。
1{
2 "Version": "2012-10-17",
3 "Statement": [
4 {
5 "Effect": "Allow",
6 "Action": [
7 "states:StartExecution"
8 ],
9 "Resource": [
10 "${Step Functionsのarn}"
11 ]
12 }
13 ]
14}
動かしてみる
ロググループがたくさんエクスポートされていきます。 ちなみに、AWSアカウント内のロググループを全てエクスポートしたら、下のようになりました。 (表示列は設定変更しています)
まとめ
今回はStep Functionsを使って、複数のCloudWatch LogsのロググループをS3にエクスポートする機能を作りました。
Lambdaを再帰的に実行する簡易なステートマシンですが、 Waitを外出ししているため、 Lambdaの実行時間制限をカバーしてくれています。
ステートマシンでは Lambdaの戻り値を使うことできる ため、 Lambda関数自体も更に分割することが可能 です。 今までの重厚なLambda関数をより視覚的にもわかりやすくすることができるのは魅力ですね。
Step FunctionsはLambda以外にも様々なAWSリソースと連携ができるので、 ステートマシンを軸としたサーバレスな事例が多く紹介されてくることでしょう。